Pygame Zero lacks a number of functions that are present in Scratch and commonly used in games. The Pygame Zero Helper library aims to remedy this, by providing the missing functions.
To use it, add from pgzhelper import *
to the top of your Python program, just after import pgzrun
. Example…
import pgzrun from pgzhelper import * alien = Actor('alien') alien.flip_x = True def draw(): alien.draw() pgzrun.go()
Functions provided by pgzhelper includes…
Actor.flip_x, Actor.flip_y
Flips the image in the x or y direction. Example…
alien = Actor('alien') alien.flip_x = True
Actor.scale
Scale the image. Takes a float. Example…
alien = Actor('alien') alien.scale = 0.5 # Half size alien.scale = 2 # Double size
Actor.move_forward(), Actor.move_back(), Actor.move_right(), Actor.move_left()
Move the actor relative to the direction it is facing. By default, the Actor is facing right. Example…
alien = Actor('alien') alien.angle = 45 alien.move_forward(50) # Moves towards the North-East
Actor.direction, Actor.move_in_direction()
Move the actor in the set direction. Unlike angle and move_forward(), this does not rotate the actor image.
alien = Actor('alien') alien.direction = 45 # Does not rotate the actor alien.move_in_direction(50) # Moves towards the North-East
Actor.distance_to(), Actor.direction_to()
Get the distance or direction from the actor to another actor.
alien = Actor('alien') hero = Actor('hero') print(alien.distance_to(hero)) # Prints the distance in pixels print(hero.direction_to(alien)) # Prints the direction in degrees
Actor.move_towards(), Actor.point_towards()
Move or point the actor towards another actor. Using move_towards will cause the actor to move without turning. Using point_towards will cause the actor to turn without moving.
alien = Actor('alien') hero = Actor('hero') hero.move_towards(alien, 5) # Moves 5 steps towards the alien actor alien.point_towards(hero) # Turn the alien towards the hero actor
Actor.get_rect()
Get the rectangle for the Actor image. Example
alien = Actor('alien') screen.draw.rect(alien.get_rect(), (255,0,0))
Actor.images, Actor.next_image()
Set a list of images. Switch the image to the next one in the list.
alien = Actor('alien_run1') alien.images = ['alien_run1','alien_run2','alien_run3'] def update(): alien.next_image() def draw(): alien.draw()
Actor.fps, Actor.animate()
Works like Actor.next_image(), but only switch image based on the specified frames per second (default is 5). Must set Actor.images first.
alien = Actor('alien_run1') alien.images = ['alien_run1','alien_run2','alien_run3'] alien.fps = 10 def update(): alien.animate() def draw(): alien.draw()
Actor.collidepoint_pixel(), Actor.collide_pixel(), Actor.collidelist_pixel(), Actor collidelistall_pixel()
Pixel perfect version of the Rect collide. Like Scratch, this ignores transparent areas when detecting collision. WARNING: This can cause poor performance and “stuck” actors. It’s usually preferable to use the normal Rect collide instead.
alien = Actor('alien') def on_mouse_down(pos): if alien.collidepoint_pixel(pos): print("Eek!")
Actor.obb_collidepoint(), Actor.obb_collidepoints()
Detect collision against a point using an oriented bounding box (obb). The normal collision detection provided by Pygame and Pygame Zero uses an axis aligned bounding box (aabb), which is fast, but works poorly when the actor is rotated. The pixel perfect collision detection is accurate, but very slow. The OBB collision detection provided here is fast and works well with a rotated actor, but only detects collision against a point. It is good for detecting collisions against bullets and mouse clicks.
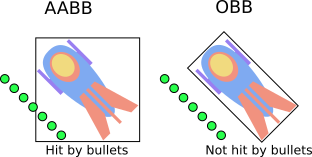
def update(): # Bullets is a list of actors hit = alien.obb_collidepoints(bullets) if hit != -1: bullets.pop(hit) print("Eek!") def on_mouse_down(pos): if alien.obb_collidepoint(pos): print("Ook!")
Actor.circle_collidepoint(), Actor.circle_collidepoints()
Detect collision using a circle with the provided radius. Depending on your image, this may give a better result than using a rectangular bounding box (eg. when your image is shaped like a circle). It’s fast, but only detects collisions against points. You can choose the collision detection radius, and it can be larger or smaller than the image.
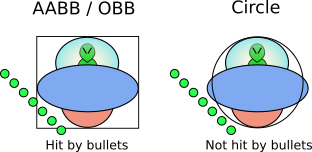
def update(): # Bullets is a list of actors # 50 is the collision detection radius hit = ufo.circle_collidepoints(50, bullets) if hit != -1: bullets.pop(hit) print("Eek!") def on_mouse_down(pos): if ufo.obb_collidepoint(50, pos): print("Ook!")
set_fullscreen(), set_windowed(), toggle_fullscreen(), hide_mouse(), show_mouse()
Does what their names suggests…
hide_mouse() # Hides the mouse cursor def update(): if keyboard.f: toggle_fullscreen() # Switch between fullscreen and windowed
More documentations available here